mirror of
https://git.openwrt.org/openwrt/openwrt.git
synced 2024-06-16 12:14:01 +02:00
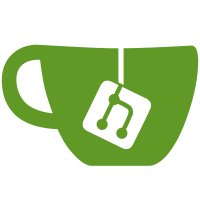
Enable testing kernel. Fix compile errors by using new kernel APIs. Fix fuzz by manually editing patches to ensure the code goes in the right place. For 721-NET-no-auto-carrier-off-support.patch, revert upstream commit a307593a6 to keep the OpenWrt ralink driver operational. Add mt7621-pci-phy patch to select REGMAP_MMIO as discussed in PR #3693 and #3952. Run automatic quilt refresh on the rest. Signed-off-by: Ilya Lipnitskiy <ilya.lipnitskiy@gmail.com>
98 lines
2.3 KiB
Diff
98 lines
2.3 KiB
Diff
--- a/drivers/misc/Makefile
|
|
+++ b/drivers/misc/Makefile
|
|
@@ -50,6 +50,7 @@ obj-$(CONFIG_GENWQE) += genwqe/
|
|
obj-$(CONFIG_ECHO) += echo/
|
|
obj-$(CONFIG_CXL_BASE) += cxl/
|
|
obj-$(CONFIG_PCI_ENDPOINT_TEST) += pci_endpoint_test.o
|
|
+obj-$(CONFIG_SOC_MT7620) += linkit.o
|
|
obj-$(CONFIG_OCXL) += ocxl/
|
|
obj-y += cardreader/
|
|
obj-$(CONFIG_PVPANIC) += pvpanic.o
|
|
--- /dev/null
|
|
+++ b/drivers/misc/linkit.c
|
|
@@ -0,0 +1,84 @@
|
|
+/*
|
|
+ * This program is free software; you can redistribute it and/or modify
|
|
+ * it under the terms of the GNU General Public License version 2 as
|
|
+ * publishhed by the Free Software Foundation.
|
|
+ *
|
|
+ * Copyright (C) 2015 John Crispin <blogic@openwrt.org>
|
|
+ */
|
|
+
|
|
+#include <linux/module.h>
|
|
+#include <linux/platform_device.h>
|
|
+#include <linux/of.h>
|
|
+#include <linux/mtd/mtd.h>
|
|
+#include <linux/gpio.h>
|
|
+
|
|
+#define LINKIT_LATCH_GPIO 11
|
|
+
|
|
+struct linkit_hw_data {
|
|
+ char board[16];
|
|
+ char rev[16];
|
|
+};
|
|
+
|
|
+static void sanify_string(char *s)
|
|
+{
|
|
+ int i;
|
|
+
|
|
+ for (i = 0; i < 15; i++)
|
|
+ if (s[i] <= 0x20)
|
|
+ s[i] = '\0';
|
|
+ s[15] = '\0';
|
|
+}
|
|
+
|
|
+static int linkit_probe(struct platform_device *pdev)
|
|
+{
|
|
+ struct linkit_hw_data hw;
|
|
+ struct mtd_info *mtd;
|
|
+ size_t retlen;
|
|
+ int ret;
|
|
+
|
|
+ mtd = get_mtd_device_nm("factory");
|
|
+ if (IS_ERR(mtd))
|
|
+ return PTR_ERR(mtd);
|
|
+
|
|
+ ret = mtd_read(mtd, 0x400, sizeof(hw), &retlen, (u_char *) &hw);
|
|
+ put_mtd_device(mtd);
|
|
+
|
|
+ sanify_string(hw.board);
|
|
+ sanify_string(hw.rev);
|
|
+
|
|
+ dev_info(&pdev->dev, "Version : %s\n", hw.board);
|
|
+ dev_info(&pdev->dev, "Revision : %s\n", hw.rev);
|
|
+
|
|
+ if (!strcmp(hw.board, "LINKITS7688")) {
|
|
+ dev_info(&pdev->dev, "setting up bootstrap latch\n");
|
|
+
|
|
+ if (devm_gpio_request(&pdev->dev, LINKIT_LATCH_GPIO, "bootstrap")) {
|
|
+ dev_err(&pdev->dev, "failed to setup bootstrap gpio\n");
|
|
+ return -1;
|
|
+ }
|
|
+ gpio_direction_output(LINKIT_LATCH_GPIO, 0);
|
|
+ }
|
|
+
|
|
+ return 0;
|
|
+}
|
|
+
|
|
+static const struct of_device_id linkit_match[] = {
|
|
+ { .compatible = "mediatek,linkit" },
|
|
+ {},
|
|
+};
|
|
+MODULE_DEVICE_TABLE(of, linkit_match);
|
|
+
|
|
+static struct platform_driver linkit_driver = {
|
|
+ .probe = linkit_probe,
|
|
+ .driver = {
|
|
+ .name = "mtk-linkit",
|
|
+ .owner = THIS_MODULE,
|
|
+ .of_match_table = linkit_match,
|
|
+ },
|
|
+};
|
|
+
|
|
+int __init linkit_init(void)
|
|
+{
|
|
+ return platform_driver_register(&linkit_driver);
|
|
+}
|
|
+late_initcall_sync(linkit_init);
|